Singleton design pattern is one of simplest design patterns. This pattern ensures that class has only one instance and provides global point of accessing it. The pattern ensures that only one object of a particular class is ever created. All further references to objects of the singleton class refer to the same underlying instance.
There are situations in a project where we want only one instance of the object to be created and shared between the clients. No client can create an instance from outside. It is more appropriate than creating a global variable as this may be copied and leading to multiple access points.
The UML class diagram below describes an implementation of the abstract factory design pattern:

In the above singleton patterns UML diagram “
GetInstance
” method should be declared as static
. This method returns single instance held in private
“instance
” variable. In singleton pattern, define all the methods and instance as static
. The static
keyword ensures that only one instance of object is created and you can call methods of class without creating object.
The constructor of class is marked as
private
. This prevents any external classes from creating new instances. The class is also sealed to prevent inheritance, which could lead to sub classing that breaks the singleton rules.
The following code shows the basic template code of the singleton design pattern implemented using C#.NET:
The eager initialization of singleton pattern is as follows:

Lazy initialization of singleton pattern:

Thread-safe (Double-checked Locking) initialization of singleton pattern:

In the above code "
lockThis
" object and the use of locking within the "GetInstance
" method. As programs can be multithreaded, it is possible that two threads could request the singleton before the instance variable is initialized. By locking the dummy "lockThis
" variable, all other threads will be blocked. This means that two threads will not be able to simultaneously create their own copies of the object.Real World Example of Abstract Factory Design Pattern using C#.NET
I am trying to apply this pattern in my application where I want to maintain application state for user login information and any other specific information which is required to be instantiated only once and held only one instance.
Class ApplicationState
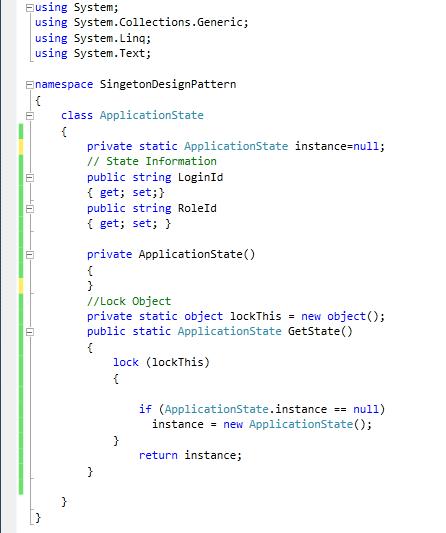
Singleton Pattern Form

OUTPUT

The above sample code creates two new variables and assigns the return value of the
GetState
method to each. They are then compared to check that they both contain the same values and a reference to the same object.
No comments:
Post a Comment