It is used to create a set of related objects or dependent objects. The “family” of objects created by factory is determined at run-time according to the selection of concrete factory class.
Abstract factory pattern acts as super factory which creates other factories. In abstract factory, interface is responsible for creating a set of related objects or dependent objects without specifying their concrete classes.
The UML class diagram below describes an implementation of the abstract factory design pattern:
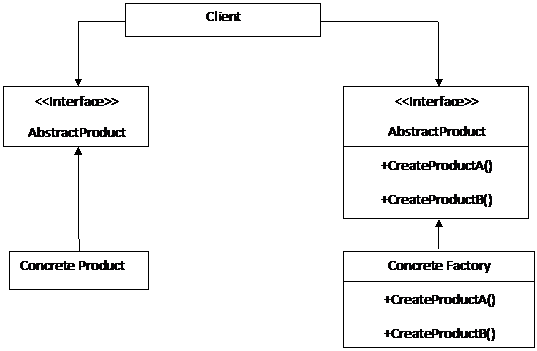
The classes,objects and interfaces used in the above UML diagram is described below:
- Client: This class is used Abstract Factory and Abstract Product interfaces to create family of related objects.
- Abstract Factory: This is an interface which is used to create abstract products.
- Abstract Product: This is an interface which is used to declare type of products.
- Concrete Factory: This is a class which implements the abstract factory interface to create concrete products.
- Concrete Product: This is a class which implements the abstract product interface to create products.
The following code shows the basic template code of the abstract factory design pattern implemented using C#.NET:


In the above abstract factory design pattern source code template client has two
private
fields that hold the instances of abstract
product classes. These objects will be accessed by inheriting their base class interface. When a client is instantiated, a concrete factory object is passed to its constructor and populate private
fields of client with appropriate data or value.
The
Abstractfactory
is base class to concrete factory classes which generate or create set of related objects. This base class contains methods definition for each type of object that will be instantiated. The base class is declared as Abstract
so that it can be inherited by other concrete factory subclasses.
The concrete factory classes are inheriting from
Abstractfactory
class and override the method of base class to generate a set of related objects required by client. There can be n number of concrete factory classes depending on software or application requirement.Abstractproduct
is the base class for the types of objects that factory class can create. There should be one base type for every distinct types of product required by client. The concrete product classes are inheriting from Abstractproduct
class. Each class contains specific functionality. Objects of these classes are generated by abstractfactory
classes to populate client.Real World Example of Abstract Factory Design Pattern using C#.NET
As an example, consider a system that does the packaging and delivery of items for a web-based store. The company delivers two types of products. The first is a standard product that is placed in a box and delivered through the post with a simple label. The second is a delicate item that requires shockproof packaging and is delivered via a courier.
In this situation, there are two types of objects required, a packaging object and a delivery documentation object. We could use two factories to generate these related objects. The one factory will be responsible for creating packaging and other delivery objects for standard parcels. The second will be responsible for creating packaging and delivery objects for delicate parcels.
Class Client

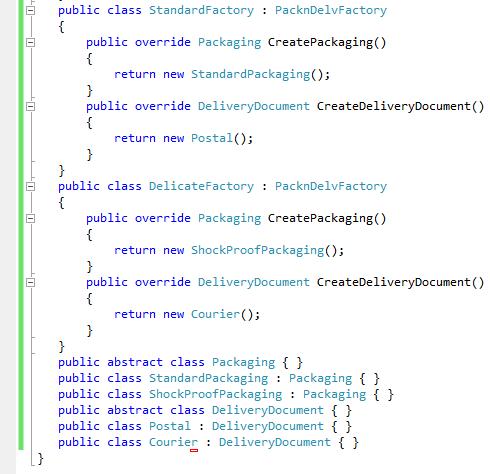
AbstractFactory Patterns Form

OUTPUT

In the above example, code creates two client objects, each passing to different type of factory constructor. Types of generated objects are accessed through the client properties.
Note
While studying abstract factory pattern, one question comes in my mind, i.e., what are concrete classes? So I Google searched the same and below is the answer to my question.
Concrete class is nothing but normal class, which is having all basic class features, like variables, methods, constructors, etc.
We can create an instance of the class in the other classes.
No comments:
Post a Comment